Algorithm Series: Exploring the Two Pointer Technique
-
Samuel Dang
- June 17, 2023
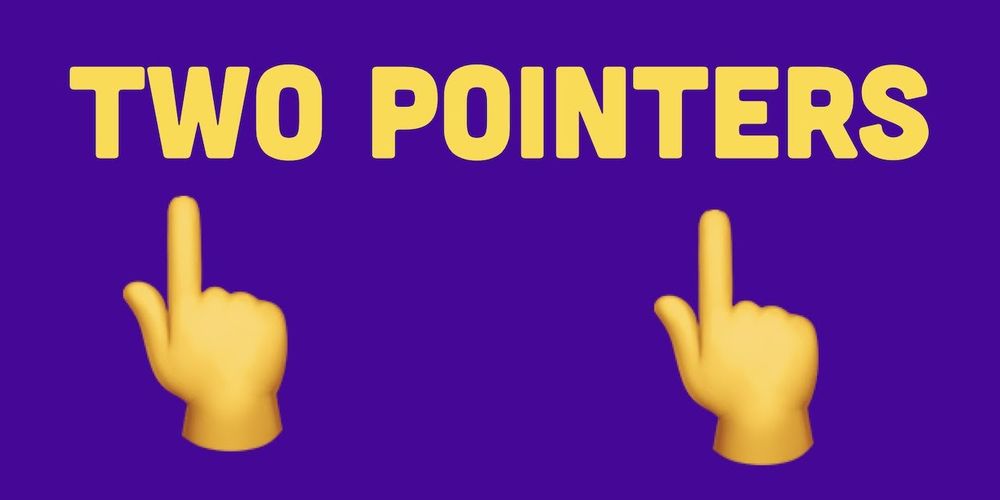
The
two pointer
technique is a powerful algorithmic approach used in various programming scenarios, especially when dealing with arrays or linked lists. It involves using two pointers to iterate through the data structure, often with different speeds or starting positions. This technique can greatly optimize time and space complexity, making it a valuable tool in problem-solving. In this article, we will explore the concept of the two pointer technique and provide clear examples implemented in JavaScript.
Understanding the Two Pointer Technique
The two pointer
technique involves maintaining two pointers, often referred to as left
and right
to traverse the given data structure simultaneously. These pointers can be moved towards each other, in the same direction, or with different speeds, depending on the specific problem requirements. By using two pointers, we can efficiently navigate through the elements and perform comparisons, modifications, or calculations.
Example 1: Two Sum Problem
Let’s consider the popular Two Sum problem as an example to demonstrate the two pointer technique. Given an array of integers, we need to find two numbers that add up to a specific target sum. Here’s a step-by-step implementation using the two pointer technique:
function twoSum(nums, target) {
let left = 0;
let right = nums.length - 1;
while (left < right) {
const sum = nums[left] + nums[right];
if (sum === target) {
return [left, right]; // Found the pair
} else if (sum < target) {
left++; // Move the left pointer towards right
} else {
right--; // Move the right pointer towards left
}
}
return []; // No valid pair found
}
const nums = [2, 7, 11, 15];
const target = 9;
console.log(twoSum(nums, target)); // Output: [0, 1]
In this example, we initialize the left pointer at the beginning of the array and the right pointer at the end. We calculate the sum of the elements pointed by the two pointers and compare it with the target sum. If the sum is equal to the target, we have found the pair. Otherwise, we adjust the pointers based on the sum’s comparison with the target and continue the iteration until we find a valid pair or exhaust all possibilities.
Example 2: Palindrome Check
Another application of the two pointer technique is in checking whether a given string is a palindrome. A palindrome is a string that reads the same forwards and backward. Here’s an implementation using the two pointer technique:
function isPalindrome(str) {
let left = 0;
let right = str.length - 1;
while (left < right) {
if (str[left] !== str[right]) {
return false; // Not a palindrome
}
left++; // Move the left pointer towards right
right--; // Move the right pointer towards left
}
return true; // Palindrome
}
const str = "racecar";
console.log(isPalindrome(str)); // Output: true
In this example, we initialize the left pointer at the beginning of the string and the right pointer at the end. We compare the characters pointed by the two pointers, moving them towards each other. If at any point the characters don’t match, the string is not a palindrome. Otherwise, if the pointers meet in the middle without any mismatch, the string is a palindrome.
Conclusion
The two pointer
technique is a powerful approach in solving various programming problems efficiently. By using two pointers to traverse data structures, we can optimize time and space complexity. In this article, we explored the concept of the two pointer
technique and provided examples using JavaScript to solve the Two Sum problem and check for palindromes. Understanding and mastering this technique will enhance your problem-solving skills and allow you to tackle a wide range of programming challenges effectively.