Algorithm Series: Divide and Conquer Technique
-
Samuel Dang
- June 18, 2023
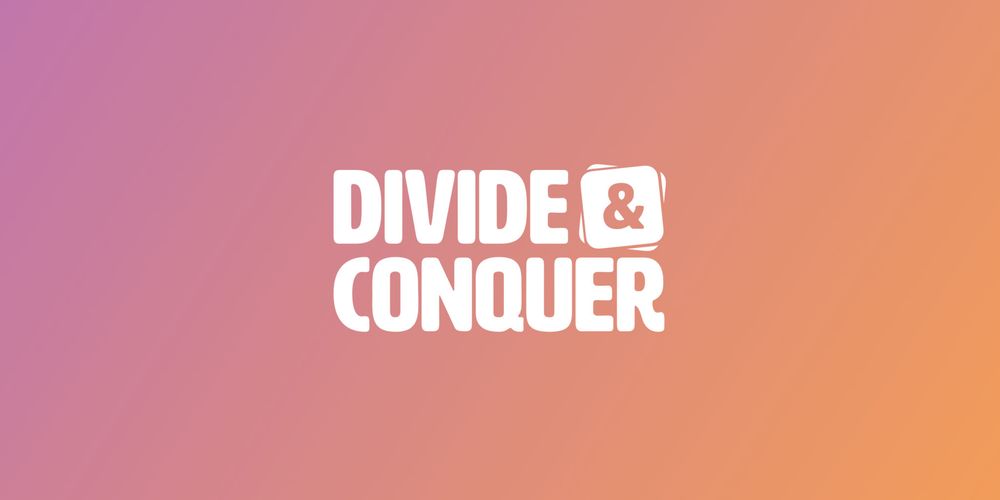
The divide and conquer algorithmic technique is a powerful approach used in computer science and mathematics to solve complex problems by breaking them down into smaller, more manageable subproblems. This technique follows the principle of dividing a problem into smaller subproblems, solving them recursively, and then combining the solutions to obtain the final result. In this article, we will explore the divide and conquer strategy in detail, discuss its advantages, and provide clear examples to illustrate its application.
Divide and Conquer Approach:
The divide and conquer approach consists of three main steps: divide
, conquer
, and combine
.
1. Divide:
In the divide step, the problem is divided into smaller, more manageable subproblems. This typically involves breaking the problem into two or more subproblems of roughly equal size. The division can be performed recursively until the subproblems become simple enough to be solved directly.
2. Conquer:
In the conquer step, each subproblem is solved independently. This is often done recursively by applying the divide and conquer technique to each subproblem. The solutions to the subproblems are obtained either by solving them directly if they are simple enough or by applying the divide and conquer approach again.
3. Combine:
In the combine step, the solutions to the subproblems are combined to obtain the final solution to the original problem. This step involves merging the subproblem solutions in a way that produces the desired result.
Benefits of Divide and Conquer:
The divide and conquer technique offers several benefits:
-
Efficient Problem Solving: By breaking down a complex problem into smaller subproblems, the divide and conquer approach simplifies the problem-solving process and allows for efficient computation.
-
Modularity and Reusability: The technique promotes modularity by encapsulating subproblems as separate entities. This modularity enables code reusability and promotes a more organized and maintainable codebase.
-
Parallelizability: Divide and conquer algorithms often lend themselves well to parallel processing, as the independent subproblems can be solved concurrently on different processors or threads, leading to potential performance gains.
Example: Finding the Maximum Number in an Array
Let’s consider an example of using the divide and conquer technique to find the maximum number in an array. Suppose we have an array of integers and we want to find the largest element in the array.
function findMax(nums) {
// Base case: If the array has only one element, return it as the maximum
if (nums.length === 1) {
return nums[0];
}
// Divide the array into two halves
const mid = Math.floor(nums.length / 2);
const leftHalf = nums.slice(0, mid);
const rightHalf = nums.slice(mid);
// Conquer: Recursively find the maximum in each half
const leftMax = findMax(leftHalf);
const rightMax = findMax(rightHalf);
// Combine: Return the larger of the two maximums
return leftMax > rightMax ? leftMax : rightMax;
}
const nums = [7, 2, 9, 4, 1, 8, 5];
console.log(findMax(nums)); // Output: 9
In this example, the findMax
function uses the divide and conquer technique to solve the problem. It divides the array into two halves and recursively finds the maximum in each half. The base case is when the array has only one element, in which case that element is returned as the maximum. The maximums from the left and right halves are then compared in the combine step, and the larger of the two is returned as the overall maximum.
By breaking down the problem into smaller subproblems and combining the solutions, the divide and conquer technique efficiently finds the maximum number in the array. The time complexity of this algorithm is O(n log n), where n is the size of the array, as it recursively divides the array into halves.
Conclusion
The divide and conquer technique is a powerful algorithmic approach that breaks down complex problems into smaller, more manageable subproblems. By dividing, conquering, and combining the solutions to these subproblems, the divide and conquer strategy enables efficient problem solving, promotes modularity and reusability, and allows for potential parallelization. The merge sort algorithm and other examples highlighted in this article demonstrate the practical application of the divide and conquer technique. Understanding and utilizing the divide and conquer approach can greatly enhance a programmer’s ability to tackle challenging problems and optimize algorithms for various applications.