Javascript Series: Introduction to Promises
-
Samuel Dang
- June 13, 2023
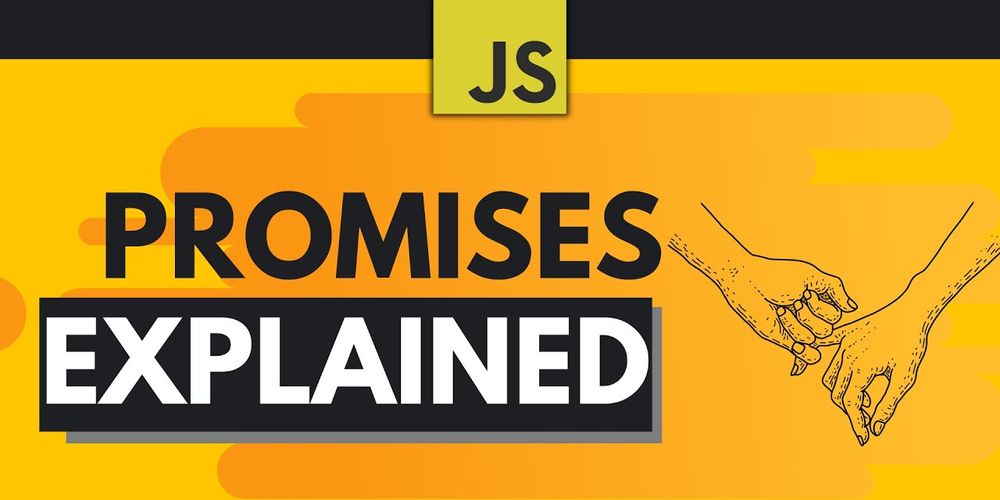
Promises are a fundamental concept in modern JavaScript for managing asynchronous operations. They provide a clean and elegant way to write asynchronous code, making it easier to handle complex tasks. In this article, we will explore the concept of promises in JavaScript and learn how to harness their power for efficient asynchronous programming.
Understanding Promises
At its core, a promise is an object that represents the eventual completion or failure of an asynchronous operation and the resulting value. It serves as a placeholder for a value that may not be available immediately but will be resolved in the future. Promises are commonly used when working with network requests, database operations, or any other tasks that involve waiting for an asynchronous response.
Creating Promises
Promises can be created using the Promise
constructor. The constructor takes a single function as an argument, known as the executor function, which is responsible for initiating the asynchronous operation. The executor function receives two parameters: resolve
and reject
. The resolve
function is called when the asynchronous operation is successful, while the reject
function is called when it encounters an error.
Here’s an example of creating a promise that simulates a network request:
const fetchData = new Promise((resolve, reject) => {
// Simulating an asynchronous network request
setTimeout(() => {
const data = { name: 'John Doe', age: 25 };
resolve(data); // Resolve the promise with the fetched data
}, 2000);
});
Chaining Promises
One of the powerful features of promises is the ability to chain them together, enabling sequential execution of asynchronous tasks. By using the then()
method, we can attach callback functions to handle the resolved value of a promise. These callback functions can also return promises, allowing for further chaining and creating a chain of asynchronous operations.
Here’s an example of chaining promises to fetch user data and then fetch additional information based on the user’s ID:
fetchUser()
.then(user => fetchAdditionalInfo(user.id))
.then(info => {
// Handle the additional information
})
.catch(error => {
// Handle any errors that occurred in the promise chain
});
Handling Errors
Error handling is an important aspect of asynchronous programming. Promises provide a built-in mechanism for error handling through the catch()
method. When an error occurs in any part of the promise chain, the control is transferred to the nearest catch()
method, allowing you to gracefully handle the error and prevent it from crashing your application.
fetchData()
.then(data => {
// Process the fetched data
})
.catch(error => {
// Handle any errors that occurred during the fetch operation
});
Promise APIs
JavaScript provides several built-in APIs that return promises, making it easier to work with common asynchronous operations. Some of these APIs include fetch()
for making HTTP requests, setTimeout()
for delaying operations, and Promise.all()
for executing multiple promises in parallel. Understanding and utilizing these APIs can greatly simplify and enhance your asynchronous programming workflow.
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => {
// Process the fetched data
})
.catch(error => {
// Handle any errors that occurred during the fetch operation
});
Conclusion
Promises are a powerful tool in JavaScript for handling asynchronous operations. They provide a structured and manageable way to deal with complex asynchronous tasks, making code more readable and maintainable. By mastering promises and understanding their intricacies, you can write efficient and robust asynchronous code that handles errors gracefully and delivers a smooth user experience. So embrace promises and unlock the full potential of asynchronous programming in JavaScript.