Javascript Series: Hoisting - Unlocking its Mysteries?
-
Samuel Dang
- June 12, 2023
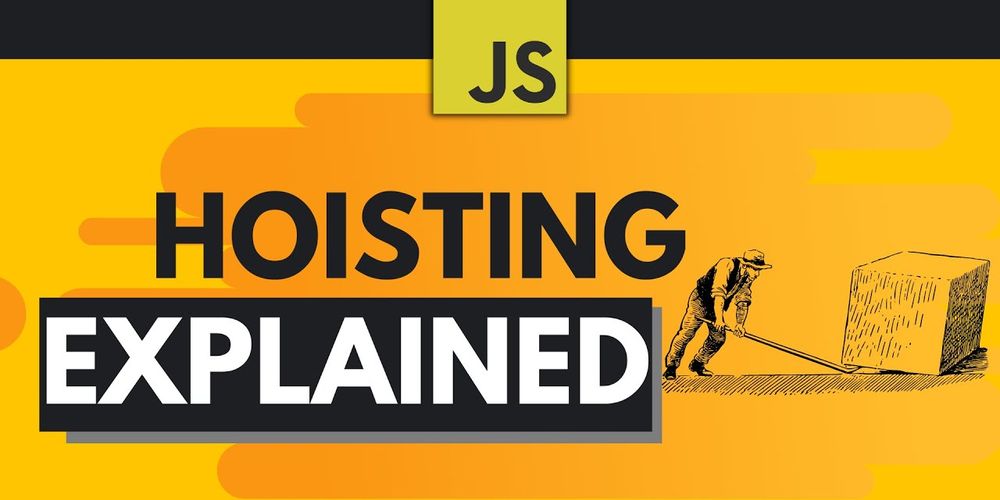
When starting to learn JavaScript, you may come across a concept called “hoisting.” This concept can be confusing and make debugging more challenging. In this article, we will explore hoisting in JavaScript and how it works.
Basic Concept of Hoisting
Hoisting is a feature in JavaScript that allows variable and function declarations to be “moved” to the top of the current scope before the code is executed. In other words, regardless of where you declare a variable or function within a scope, they will be moved to the top of the scope before the program runs.
Variable Hoisting
In JavaScript, when you declare a variable using the var keyword, it will be hoisted to the top of the current scope. This means that you can access the variable before it is declared.
console.log(name); // Output: undefined
var name = "John";
In the above example, the variable name
is declared after accessing it. However, JavaScript will hoist the variable name
to the top of the scope and assign the value undefined
to it. Therefore, when running console.log(name)
, the result will be undefined
.
Hoisting with Functions
The concept of hoisting also applies to functions in JavaScript. When you declare a function, it will also be hoisted to the top of the current scope.
sayHello(); // Output: "Hello, there!"
function sayHello() {
console.log("Hello, there!");
}
In the above example, we call the sayHello() function before declaring it. However, the function is hoisted before the code runs, so the result will be “Hello, there!“.
Hoisting does not apply to let and const
It is important to note that hoisting only applies to variables declared with var and does not apply to let and const.
console.log(age); // Output: ReferenceError: age is not defined
let age = 30;
In the above example, when accessing the variable age before declaring it, JavaScript throws a “ReferenceError” because age has not been declared. This occurs because let and const are not hoisted to the top of the scope.
Conclusion
JavaScript hoisting is an important feature to understand when working with the language. It is crucial to remember that only variable declarations with the var keyword and function declarations are hoisted. Variables declared with let and const are not hoisted, and accessing them before declaration will result in an error. Understanding hoisting will help you write more accurate and understandable code in JavaScript.
We hope this article has helped you gain a better understanding of hoisting in JavaScript. Stay tuned to our blog to learn more about other important concepts and techniques in the programming field!